You are here reading this article means you are stunned by the behavior of float values in PHP.
You might wonder why a separate article on this simple topic but before thinking that try running the below php code once.
echo (5.63+0.06) - 5.69;
Are you surprised now? You will end up with the result like -8.8817841970013E-16 even if we have the same value at both sides of expression.
Before we look into the correct way to deal with float values in PHP, first have a look at the reason for this odd behaviour of float values in PHP.
PHP official website has already added a warning for the same behaviour of float values. So I will grab that exact explanation here:
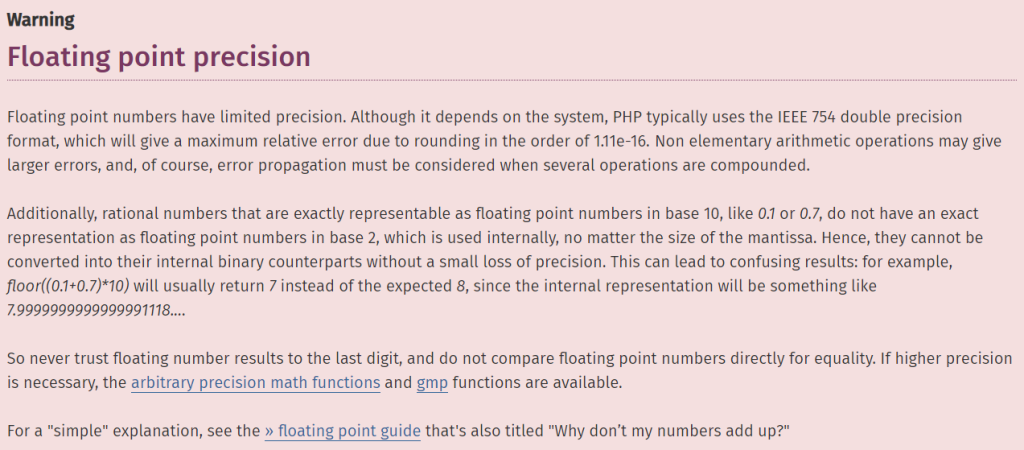
Up to here, we have seen why this type of strange behavior happens while working with float values in PHP. Now time to see how we can deal with these float values in PHP.
Comparing float values in PHP
First, we will see how you can get into a trap of float values while comparing those. We will with code examples to understand this in a better way. Refer few code blocks below to check the same:
$a = 5.69;
$b = 5.69;
var_dump($a == $b);
// Output: bool(true)
In the above code block, we can see that both values are the same and giving us the proper result. Now let’s check the tricky part in comparing float values in PHP.
$a = 5.63 + 0.06;
$b = 5.69;
var_dump($a == $b);
// Output: bool(false)
Solution
A quick and simple solution to this problem in comparing float values is to cast that to string and compare those values. Now it will compare two strings and it does not have any precision error.
$a = (string) (5.63 + 0.06);
$b = (string) 5.69;
var_dump($a == $b);
// Output: bool(true)
If you think (like me) converting to a string gives us a correct result. But is not an ideal solution then the next solution is for you.
To get the solution of the above problem, we will need BCMath PHP Extension installed with PHP. You can read this article on how to install PHP BCMath in PHP.
Once you are done with installing BCMath extension, you can use below code and get the proper result. Instead of using direct sum we will use the bcadd method.
$a = bcadd(5.63, 0.06, 2);
$b = bcadd(5.69, 0, 2);
var_dump($a == $b);
// Output: bool(true)
Let’s have a look at one more example of the same kind of case:
echo bcadd(5.63, 0.06, 2) - 5.69;
You can find all the methods available for BCMath here. http://php.net/manual/en/ref.bc.php
Share if you have a similar kind of experience working with float values in PHP.
Really? This issue has existed since programmers advanced beyond machine language. Never compare two numbers! Instead, test to see if their absolute difference is below some reasonable threshold. |a-b|<$threshold will never let you down, regardless of implementation or high-level language.